After I got some feedback and new ideas from the GitHub repository, I implemented the following features for the 1.6.0 build. Additionally, I finally rewrote most of the logic of the SquareProgressBar drawing method. Together with a new icon and some changes to the UI, I just released the new major version 1.6.0. Get it via gradle:
dependencies {
compile 'ch.halcyon:squareprogressbar:1.6.0'
}
indeterminate feature
Suggested on GitHub (issue #26) was an indeterminate ProgressBar that always runs around the image to visualise a progress for which we don’t know the final duration or the current progress. You still can customise the SquareProgressBar with colours, size, opacity and so on. All these settings still work with this indeterminate ProgressBar. To activate this, simply set the following flag on the SquareProgressBar :
squareProgressBar.setIndeterminate(true)
After this is set the SquareProgressBar will behave like shown in the following video:
center line feature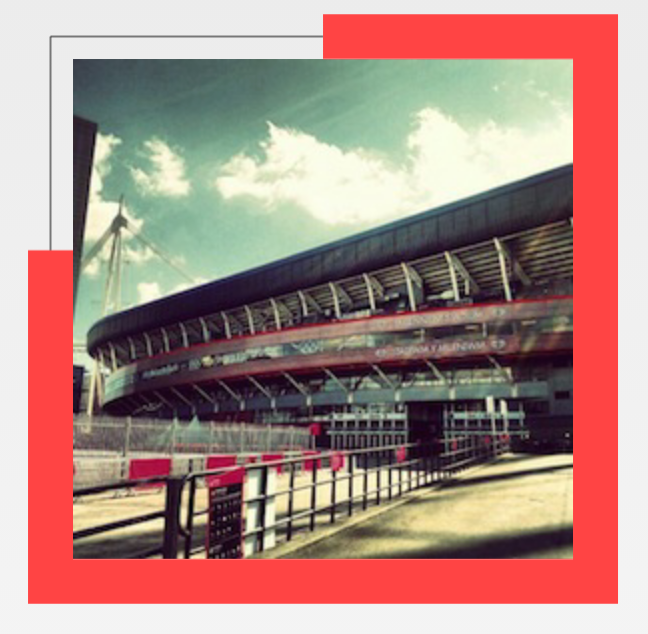
This is a very small addition to the style settings. It simply displays a small line in the middle of the path that the SquareProgressBar has to go. You can simply set this via the following line of code:
squareProgressBar.drawCenterline(true)
You can see an example of the center line setting on the right side.
getImageView
Another request (issue #34) was to provide a getter method for the ImageView. There are android-libraries that need the ImageView to do some async loading of the images or something else. So this is available now as well on the SquareProgressBar class:
/** * Returns the {@link ImageView} that the progress gets drawn around. * * @return the main ImageView * @since 1.6.0 */ public ImageView getImageView(){ return imageView; }
small changes
There are also some smaller changes to the UI. For example, there is a new image that is considerably bigger than the others in the example application. But this isn’t shown in the application anymore because the bug where the big images make the SquareProgressBar behave strangely is finally resolved. I already fixed part of the problem back in 2014, but I recently came across the right XML-setting to remove the blank spaces around an ImageView, when the Image is wider than the screen of the phone. You can see the change here commit: 513b45b. The trick was to add the following line to the XML:
android:adjustViewBounds="true"
Future changes
One thing that I want to make much simpler in the next version is the usability of text in the SquareProgressBar. I already have a good idea on how I want to achieve that, but that has to wait until I finished some other projects.
You can find the repository on GitHub as usual: android-square-progressbar
The library is available on Bintray: squareprogressbar
Recent Comments